The Ultimate Guide to Flutter State Management
Flutter has become incredibly popular in the field of mobile app development thanks to its capacity to create appealing and powerful applications. The maintenance and updating of the application’s data is a key function of state management, which is a critical component of the creation of Flutter apps. This in-depth guide will examine various state management strategies in Flutter and provide you with insightful advice on how to enhance the performance of your app.
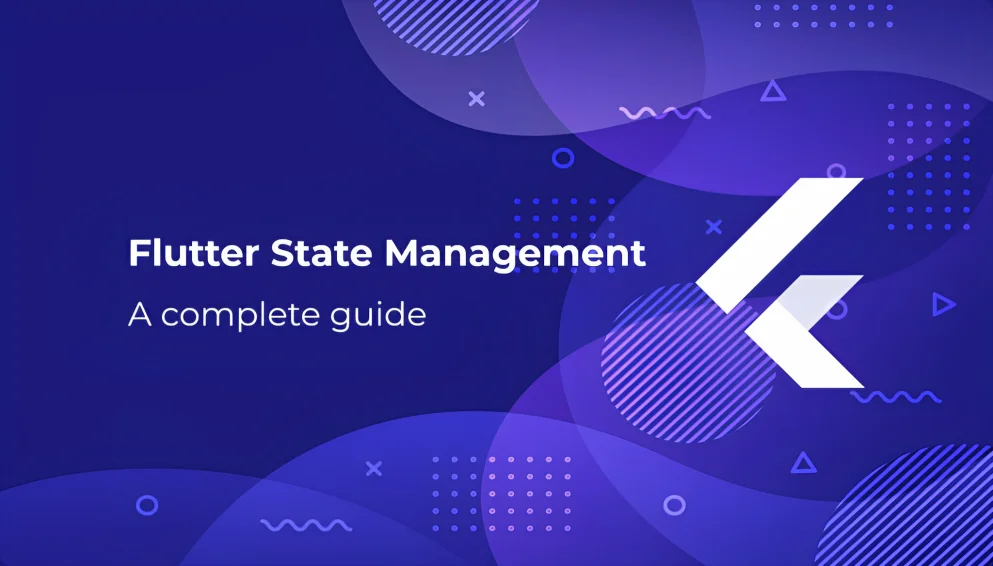
What is State Management?
Let’s first define state before delving into the mechanics of Flutter State Management. Simply put, the state of an application is a representation of the data or information that evolves. On the other hand, state management describes the methods and patterns used to manage, update, and synchronize this data across various application components.
Why is State Management Important?
For Flutter apps to be scalable and responsive, effective state management is essential. Managing an app’s state gets more difficult as it becomes more complex. A seamless user experience, efficient resource consumption, and accurate data reflection are all benefits of effective state management. You can increase the maintainability of your code and the performance of your app as a whole by selecting the appropriate state management strategy.
State Management Techniques in Flutter
StatefulWidget and setState
Using the StatefulWidget and setState methods is the most basic method of managing the state in Flutter. A widget subclass that can store mutable states is called StatefulWidget. When the state has to be updated, setState is called, which causes the widget subtree to be rebuilt and displays the new state in the user interface.
Provider
A well-liked state management library in Flutter called the provider makes maintaining the state across many widget hierarchies easier. To transmit the state farther down the widget tree, it takes advantage of the InheritedWidget notion. The provider provides a variety of providers, such as ChangeNotifierProvider, StreamProvider, and FutureProvider, to meet diverse state management needs.
BLoC (Business Logic Component) Pattern
Another popular method of state management in Flutter is the BLoC pattern. It entails isolating the business logic from the user interface elements to improve testability and reuse. Streams and sinks are used to manage states and events in the BLoC pattern. Additional functionality is offered by libraries like rxdart to simplify the BLoC pattern implementation.
Redux
A predictable state management library called Redux, which was first used in online development, has also become popular in Flutter. The state is kept in a centralized store and the architecture is one of unidirectional data flow. Reducers are used to update the state based on actions that are dispatched to modify it. Redux offers a clear mechanism for controlling intricate application states.
Riverpod
The hooks in Provider and Flutter served as inspiration for Riverpod, a provider-based state management solution. It emphasizes performance, scalability, and simplicity. You can create and consume providers using a combination of providers, consumers, and hooks using Riverpod’s versatile and modular state management system.
Choosing the Right State Management Approach
The best state management option to choose relies on several variables, including the complexity of your app, the size of your team, and your tastes. Flutter offers a variety of state management alternatives. Before choosing a course of action, it is crucial to weigh the advantages and disadvantages of each option. Take into account elements like usability, performance consequences, readable code, and community support.
Taking Your Flutter State Management to the Next Level
Now that your knowledge of Flutter state management is solid.
There are various ways you can advance your abilities.
Performance Optimisation
The performance of your state management implementation should be optimized. Investigate methods like selective widget rebuilding, employing value listeners in place of stream builders where appropriate, and making use of cache systems to reduce pointless updates. You can make Flutter applications even faster and more effective by optimizing state management performance.
Advanced State Management Patterns
Explore Flutter’s complex state management patterns to learn more. Dive deeper into methods that offer different ways to manage states in complex applications, such as a scoped model, get_it, or fish_redux. These patterns provide various viewpoints and can be useful additions to your toolset for state management.
Hybrid State Management
To maximize the benefits of different state management strategies, think about combining them. For example, you can use Redux to manage the overall state of the application, the BLoC pattern to handle business logic and data flow, and the Provider to manage a small, localized state. You may design a flexible and scalable architecture for your Flutter applications by utilizing hybrid state management.
Testing and Debugging
Learn the tricks of the testing and bug-fixing trade for state management implementations. Make sure that your state transitions and updates function as intended by creating thorough unit tests for your state management classes. Utilise Flutter’s debugging tools, such as the Flutter Inspector, to examine and keep an eye on the state changes as they happen. You may build more robust and reliable applications by using efficient testing and debugging techniques to quickly discover and fix problems.
Contributing to the Flutter Ecosystem
By sharing your state management expertise and participating in open-source projects, you can consider enhancing the Flutter ecosystem. State management software packages can benefit from lessons, sample projects, and bug fixes. You may develop your abilities and aid others in their quest to master Flutter state management by actively contributing to the community.
Best Practises for Flutter State Management
Effective implementation of the various state management approaches is just as important as comprehending them.
Here are some guidelines to follow when utilizing Flutter state management.
Identify the Scope of State
Consider the size of your state carefully before choosing a management strategy. Ascertain whether it needs to be shared across various widgets or localized to a single widget. You may select the best strategy with the aid of this analysis, which will also help you keep your code from becoming overly complex.
Minimize the Use of setState
While the setState approach is simple and appropriate for maintaining simple states, complex applications may find it burdensome. Do not overuse setState since this may result in frequent widget rebuilds. For better performance and code organization, think about using more cutting-edge state management libraries like Provider or Riverpod.
Separate UI and Business Logic
It is advised to isolate the UI components from the business logic to improve code readability and maintainability. Place the state-related business logic in distinct classes or blocks, such as BLoCs or Redux reducers, to adhere to the idea of separation of concerns. Better code reuse and testability are made possible by this separation.
Optimise State Updates
Flutter has to perform better to deliver a seamless user experience. Be careful to avoid needless rebuilds when updating the state. Use methods like memoization, where a function’s result is cached based on its input, to reduce the need for further computations and widget rebuilds.
Leverage Asynchronous State Management
Asynchronous tasks like network requests or database queries are needed by many applications. Make sure the state management strategy you select easily accommodates asynchronous state updates. Asynchronous data management methods like using Futures or Streams can keep your UI responsive while managing asynchronous data.
Monitor and Debug State changes
It is crucial to adequately monitor and troubleshoot state changes during development. Use Flutter’s development tools and debugging features to examine how state updates spread through the widget tree. By doing so, you can spot possible problems or bottlenecks and adjust how state management is implemented.
Stay Updated with the Flutter Community
The Flutter ecology is alive and changing all the time. By subscribing to the Flutter community, you can keep up with the newest developments and trends in state management. Participate in forums, go to conferences or meetups, and look into open-source libraries to learn new strategies for effective state management.
Scaling State Management for Larger Flutter Applications
Scaling your state management becomes essential as your Flutter applications get bigger and more complicated.
The following are some approaches to take into account while dealing with state management in bigger projects.
Modularization
Your application should be divided up into smaller, modular parts. Better encapsulation and maintainability are made possible by allowing each module to have its state management implementation. This strategy makes it simpler to understand the behavior of your application and lowers the complexity of managing a single global state.
Dependency Injection
To deliver instances of state management classes to various portions of your application, think about using dependency injection. This permits flexible coupling and facilitates, if necessary, the replacement or switching between various state management technologies. Get_it and Kiwi are two well-liked dependency injection frameworks for Flutter.
State Persistence
You might occasionally need to maintain your application state during device restarts or session changes. Use database frameworks like sqflite or moor or local storage options like shared_preferences to store and retrieve state information. This guarantees that users can continue using the program without interruption even after closing and reopening it.
Performance Monitoring
Keep an eye on how well your state management implementation is working to see any potential problems or potential improvement areas. Utilize the Dart Observatory and Performance Overlay performance profiling tools offered by Flutter to examine the impact of your state management on application performance. Reduce the number of unneeded rebuilds in your code, and if you can, use fewer resources.
Code Generation
To automatically construct immutable state classes and related serialization and deserialization logic, take into consideration using code generation tools such as built_value or freeze. When working with complicated state systems, these packages can minimize boilerplate code and provide type safety.
Team Collaboration
When working on state management in larger projects, successfully collaborate with your team. To maintain uniformity across the codebase, establish defined rules, coding standards, and documentation procedures. Establish recurring code reviews and promote open dialogue to address any problems or difficulties that surface during the state management implementation.
By utilizing these techniques, you can efficiently manage state in larger Flutter apps and guarantee speed as your projects expand while also ensuring scalability, maintainability, and flexibility.
Additional Resources for Flutter State Management
To improve your understanding of and competency with Flutter state management.
Explore the following supplementary resources.
Flutter Official Documentation
State management approaches are thoroughly explained and illustrated in the official Flutter documentation, which also includes tutorials and case studies. When working with Flutter state management, it is a useful resource to consult.
Flutter Community
To interact with the Flutter community, take part in online forums, discussion boards, and social networking sites. Ask questions, share your experiences, and gain knowledge from other Flutter developers’ experiences. The community is a fantastic resource for exchanging expertise and insights.
Flutter State Management Packages
Investigate the different state management tools that the Flutter ecosystem has to offer. These bundles offer ready-to-use solutions and can act as a jumping-off point for your state management installation. Riverpod, Bloc, Redux, and Provider are a few of the more well-liked packages.
Online Courses and Tutorials
Learn about Flutter State management by enrolling in online classes or by following tutorials. Various state management approaches are covered in a wide range of courses and tutorials available on websites like Udemy, Coursera, and YouTube that are geared toward different skill levels.
Open-Source Projects
Research state management approaches in open-source Flutter projects and participates in them. You can learn from seasoned developers’ best practices and obtain useful insights by looking at real-world solutions.
Flutter Meetups and Conferences
Attend regional conferences and meetups devoted to Flutter development. These gatherings offer chances to socialize with other Flutter fans, hear from industry leaders, and take part in state management-focused workshops or hackathons.
You may improve your knowledge of Flutter state management and keep up with new developments in the industry by using these resources. Do not be afraid to apply your knowledge to practical projects and experiment with various state management strategies because practice and practical experience are essential to learning any skill.
Conclusion
We have investigated numerous methods and recommended procedures to efficiently manage state in Flutter applications in our comprehensive guide to Flutter state management. StatefulWidget and setState, Provider, the BLoC pattern, Redux, and Riverpod are five common state management options that we looked at as we addressed the significance of state management for creating scalable and responsive apps.
The choice will depend on various elements, including the complexity of your software, the size of your team, and personal preferences. Each state management technique has advantages and disadvantages. You can deploy a state management solution that meets the needs of your project by making an informed decision and by comprehending the underlying concepts and patterns of each approach.
State management is not a universally applicable approach. It necessitates a comprehensive analysis of the state requirements for your app, careful evaluation of the trade-offs, and ongoing improvement. You will hone your abilities and strike a balance between simplicity, performance, and maintainability as you acquire expertise with Flutter and state management.
You can build Flutter applications that provide a seamless user experience, optimize resource usage, and handle complicated state transitions with ease by using smart state management. To stay on top of Flutter state management, keep an open mind, experiment with new libraries and methods, and stay involved in the active Flutter community.
It is now up to you to use these ideas and methods in your own Flutter projects. Create exceptional apps that stand out in the crowded market for mobile applications by experimenting, iterating, and optimizing your state management implementation.
Happy coding, and may effective state management help your Flutter applications succeed!