How to Use Firebase with Flutter for Authentication, Database, and Storage
Flutter has become incredibly popular in recent years as a cross-platform framework for creating mobile applications. It is a popular option among developers due to its comprehensive feature set and simplicity of usage. Firebase emerges as a strong and flexible option for connecting backend services with Flutter.
The capacity to build dynamic, interactive applications that deliver a seamless user experience is unlocked when you mix Firebase’s strength with Flutter’s flexibility. Firebase may significantly improve the usability and efficiency of your program, whether you are developing a social media platform, an e-commerce application, or a productivity tool.
By using Firebase Authentication, you can make sure that only authorized users can access your app, protecting user accounts and important data. You can provide users with many alternatives for securely logging in and registering thanks to the diversity of authentication methods available, such as email or password, social media sign-in, and phone number verification.
Your Flutter app can show live updates and changes as they happen with the help of Firebase Realtime Database’s real-time data synchronization feature. Applications that need chat capabilities in real-time, live data dashboards, or collaborative features would benefit the most from this capability. You may design flexible and engaging user interfaces using the Firebase Realtime Database.
Managing user-generated material, including documents, videos, and photographs, is made incredibly easy using Firebase Cloud Storage. By offering safe and expandable storage, it makes sure that your app can meet the rising demands of user-generated content. Your Flutter app can simply upload, retrieve, and serve files to users by integrating Firebase Cloud Storage, which will improve their overall experience.
Your Flutter app can benefit from additional services that Firebase provides. You can communicate with your users even when they are not actively using your app thanks to Firebase Cloud Messaging. You can use Firebase Analytics to gain an insightful understanding of user behavior, which will help you make informed decisions and improve the functionality of your app. With Firebase Remote Config, you can remotely modify a few app settings without having to update the app, providing you with more flexibility and control.
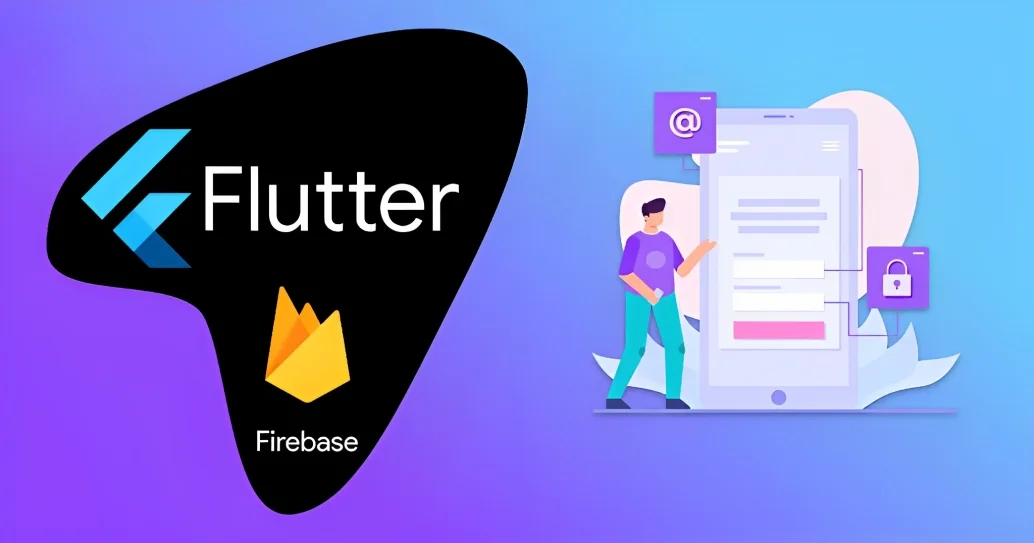
Getting Started With Firebase
You must first establish a Firebase project and make the appropriate modifications to start utilizing Firebase with Flutter. Go to the Firebase website (firebase.google.com) and log in using your Google account to get started. To configure Firebase for your Flutter application, create a new project and adhere to the on-screen instructions.
Authenticating Users
Most applications depend heavily on authentication. Firebase offers a user-friendly authentication mechanism that accepts several sign-in options, including Facebook, Google, and email or password.
Use these instructions to implement user authentication in your Flutter application.
In your Flutter project, install the Firebase Authentication package.
Install the required packages, then start Firebase.
Set up the preferred authentication option, such as social network sign-in or email and password.
Utilize the Firebase Authentication APIs to implement the authentication process in your Flutter application.
You can manage user registration, login, and password reset functionality within your Flutter app with ease by using Firebase’s authentication features.
Real-time Database Management
Firebase Realtime Database, a real-time NoSQL database offered by Firebase, enables you to store and synchronize data across several clients in real time.
Follow these steps to combine the Firebase Realtime Database with Flutter.
The Firebase Database package should be installed in your Flutter app.
Firebase should be started after importing the necessary packages.
To specify access rights, configure the database rules.
To retrieve and update data from the database, implement read and write operations.
To get updates whenever the data changes, use Firebase’s real-time listeners.
You can quickly and easily create chat applications, real-time dashboards, and collaboration capabilities for your Flutter project with the Firebase Realtime Database.
Cloud Storage Integration
Firebase offers a scalable and secure cloud storage solution called Firebase Cloud Storage for storing and serving user-generated content.
Use these procedures to integrate Firebase Cloud Storage into your Flutter application.
Your Flutter project should now contain the Firebase Storage package.
Firebase should be started after importing the necessary packages.
Create the required security policies to limit access to files that are saved.
Utilize the Firebase Cloud Storage APIs to implement file upload and download capabilities.
Use the URL generation feature of Firebase Storage to serve the files that are stored in your app.
Your Flutter application can easily manage media files, documents, and other user-generated content thanks to Firebase Cloud Storage.
Firebase Authentication: A Secure User Experience
A reliable and secure method of managing user authentication in your Flutter app is provided by Firebase Authentication. You can make sure that only authorized users can access the features and data in your app by utilizing Firebase’s authentication services. In addition to email and password authentication, Firebase also provides phone number authentication, social network sign-in options including Google, Facebook, and Twitter, and other authentication methods.
The required packages must be integrated, and the needed sign-in procedures must be configured, to implement Firebase Authentication in your Flutter application. You can handle user registration and login operations, as well as add extra features like account verification and password reset capability. You may also customize the authentication flow. You can offer a seamless and secure user experience while protecting the data in your app using Firebase Authentication.
Firebase Realtime Database: Real-Time Data Synchronisation
Realtime Database, a NoSQL cloud-hosted database that enables real-time synchronization of data across many devices, is one of Firebase’s distinguishing features. Your Flutter app can keep a synchronized and up-to-date view of data using the Firebase Realtime Database, making it perfect for collaborative applications, chat features, and real-time changes.
Installing the required packages, starting Firebase, and configuring the database access rules are all steps in integrating Firebase Realtime Database with Flutter. Then, using Firebase’s built-in listeners, you can perform read-and-write operations to get data out of the database and update it while also listening for updates in real time. With the help of this potent combo, you can build interactive and responsive features for your app that respond quickly to database changes.
Firebase Cloud Storage: Securely Store User-Generated Content
A scalable and safe option for hosting and delivering user-generated content in your Flutter app is Firebase Cloud Storage. Firebase Cloud Storage offers a dependable platform for safely storing and easily retrieving files, whether they be documents, movies, photos, or any other type of file.
You must install the appropriate packages, launch Firebase, and set up the required security rules to restrict access to the saved files to integrate Firebase Cloud Storage into your Flutter project. Then, you can include functions like file upload and download support, create URLs for the files you store in Firebase Storage to serve them in your app, and even add extra security safeguards like user-based permissions or encryption.
You can guarantee that the user-generated material in your app is securely kept and readily available by utilizing Firebase Cloud Storage, giving your users a seamless and interesting experience.
Maximizing Performance and Scalability
Your Flutter app’s efficiency and scalability can be improved even further using a variety of extra services provided by Firebase. For instance, Firebase Cloud Messaging gives you the ability to inform and engage your app’s users with tailored notifications. You may use Firebase Analytics to gain insightful information about user behavior and app performance to guide your data-driven decisions. Additionally, without the need for an app update, Firebase Remote Config enables you to dynamically modify your app’s behavior.
You may enhance performance, customize user experiences, and keep your app flexible and adaptive to changing requirements by investigating these extra Firebase services and integrating them into your Flutter project.
You may create robust and scalable applications using the full range of services offered by Firebase, which interact with Flutter without any issues. You can speed up development and provide top-notch user experiences by making use of Firebase’s functionalities.
Let’s examine a few more advantages.
Built-in Security
For the protection of your app and user data, Firebase provides strong security measures. While Firebase Realtime Database and Firebase Cloud Storage have built-in security rules to govern access to your data and files, Firebase Authentication ensures secure user login and registration processes. You can concentrate on developing your app while Firebase takes care of the security concerns.
Real-time Updates
Changes made to the data are instantly synced across all linked devices while using the Firebase Realtime Database. Collaboration tools, chat programs, and real-time updates are made possible by this real-time synchronization in your Flutter app. With no need to manually refresh the app, users can notice updates instantly, making for a seamless and engaging experience.
Scalability
A wide range of application sizes can be handled by Firebase. Whether you are developing a modestly sized app or a huge business solution, Firebase can scale easily to suit your requirements. To keep your app responsive and performant, Firebase’s architecture automatically handles traffic peaks and adjusts resources as necessary.
Serverless Architecture
Management of the backend infrastructure is not necessary thanks to Firebase. With Flutter, you can concentrate on front-end development while Firebase handles server-side operations. You may launch your app more quickly because of the time and resource savings provided by this serverless architecture.
Easy Integration
Flutter-specific packages are available from Firebase, which offers a smooth connection with Flutter. The well-documented APIs and simple examples provided by the Firebase packages make it simple for developers to integrate Firebase services into their Flutter projects.
Analytics and Monitoring
Understanding how people engage with your app is made possible with Firebase Analytics, which offers insightful data on user behavior. To make informed decisions and improve the performance of your app, you may monitor user engagement, retention rates, and conversion metrics. To monitor and track app crashes and rapidly discover and fix problems, Firebase Crashlytics is another tool you may use.
Offline Support
Because of Firebase’s offline features, your app will continue to work even if there is no network connectivity. Users can continue using some functions when offline thanks to Firebase SDKs’ local data caching capabilities. When the network connection is established again, Firebase immediately updates the server with any local modifications.
Easy Deployment
Your Flutter app’s deployment procedure is made simpler with Firebase. Using a single codebase and Firebase, you can quickly release your app to a variety of platforms, such as Android, iOS, and the web. It is really simple to build and distribute your app to a worldwide audience thanks to Firebase, which takes care of the necessary infrastructure and offers hosting services.
Rich Ecosystem
The developer ecosystem in which Firebase is embedded is thriving and active. For developers, it makes it simpler to understand and solve any problems they may run into by providing copious documentation, tutorials, and community help. The Firebase community’s knowledge and experience can help you overcome obstacles and enhance the way you create apps.
Continuous Improvement
Every day, Firebase adds new features and improves existing ones. You may benefit from these upgrades as a developer utilizing Firebase with Flutter to improve the functionality of your app and keep up with the most recent developments in mobile app development. You have access to cutting-edge tools and services thanks to Firebase’s dedication to innovation.
Cost-Effective Solution
You can start utilizing Firebase without making a sizable financial commitment because of Firebase’s variety of price tiers, which include a generous free tier. As your app becomes more popular, you can scale it up and pick a pricing strategy that suits your requirements. Because you only pay for the services you use using Firebase’s pay-as-you-go model, developers may use it without incurring excessive costs.
Third-Party Integrations
Your Flutter app’s functionality can be increased thanks to Firebase’s smooth integration with a variety of third-party services and tools. To expand the functionality of your app and learn more about user behavior and performance, you may link Firebase with well-known services like Google Cloud Platform, Crashlytics, Google Analytics, and others.
You can build high-quality, feature-rich applications that are effective, scalable, and user-friendly by taking advantage of Firebase’s robust features in combination with Flutter’s cross-platform capabilities.
Conclusion
In this post, we looked at how to use Flutter and Firebase to take advantage of Firebase’s capabilities for storage, database management, and authentication. You can successfully integrate Firebase into your Flutter app and give it user authentication, real-time database functionality, and scalable cloud storage capabilities by following the methods indicated above. You can develop feature-rich, dynamic applications that astonish your users by combining the freedom of Flutter with Firebase’s powerful features.
Keep in mind that Firebase provides a wide range of other features, including cloud messaging, analytics, and remote configuration, all of which can improve your Flutter project. To fully utilize Firebase and provide excellent user experiences, continue learning and playing with it.
So, get started on your path to creating amazing Flutter applications that are powered by Firebase.